Today I installed the GitLab Community Edition on Docker. It takes me some time to figure out how to get it done in an reasonable way. On the GitLab pages there is an installation guide for Docker. And the Docker images for GitLab are very well maintained on DockerHub. In the following I will expalin how to run GitLab with Docker-Compose and separate Database Containers.
Continue reading “Running GitLab on Docker”How to configure Security in Open Liberty Application Server?
I started to run our Imixs-Workflow engine on OpenLiberty Application Server. One important thing in Imixs-Workflow is the authentication against the workflow engine. In Open Libertry, security can be configured in the server.xml file. But it takes me some time to figure out the correct configuration of the role mapping in combination with the @RunAs annotation which we use in our service EJBs.
Continue reading “How to configure Security in Open Liberty Application Server?”Wildfly 16.0.0 with Microprofile Metrics 2.0.0
Wildfly Server is supporting the Eclipse Microprofile Metric API since version 14.0.0 . Within the Imixs-Workflow Project we started since version 5.0.0 to support Microprofile 2.0.
Continue reading “Wildfly 16.0.0 with Microprofile Metrics 2.0.0”Microprofile – Metric API: How Create a Metric Programatically
The Microprofile Metric API is a great way to extend a Microservice with custom metrics. There are a lot of examples how you can add different metrics by annotations.
@Counted(name = "my_coutner", absolute = true,
tags={"category=ABC"})
public void countMeA() {
...
}
@Counted(name = "my_coutner", absolute = true,
tags={"category=DEF"})
public void countMeB() {
...
}
In this example I use tags to specify variants of the same metric name. With MP Metrics 2.0, tags are used in combination with the metric’s name to create the identity of the metric. So by using different tags for the same metric name will result in an individual metric output for each identity.
But in case you want to create a custom metric with custom tags you can not use annotations. This is typically the case if the tags are computed by application data at runtime. The following example shows how you can create a metric with tags programatically:
...
@Inject
@RegistryType(type=MetricRegistry.Type.APPLICATION)
MetricRegistry metricRegistry;
...
Metadata m = Metadata.builder()
.withName("my_metric")
.withDescription("my description")
.withType(MetricType.COUNTER)
.build();
Tag[] tags = {new Tag("type","ABC")};
Counter counter = metricRegistry.counter(m, tags);
counter.inc();
...
Eclipse – UTF-8 Encoding
When developing a web application encoding is an important issue. Usually UTF-8 encoding should be the best choice if you need to implement multi-lingual web application.
However in Eclipse there is a default setting of ISO-8859-1 encoding for so called ‘Java Property Files’.
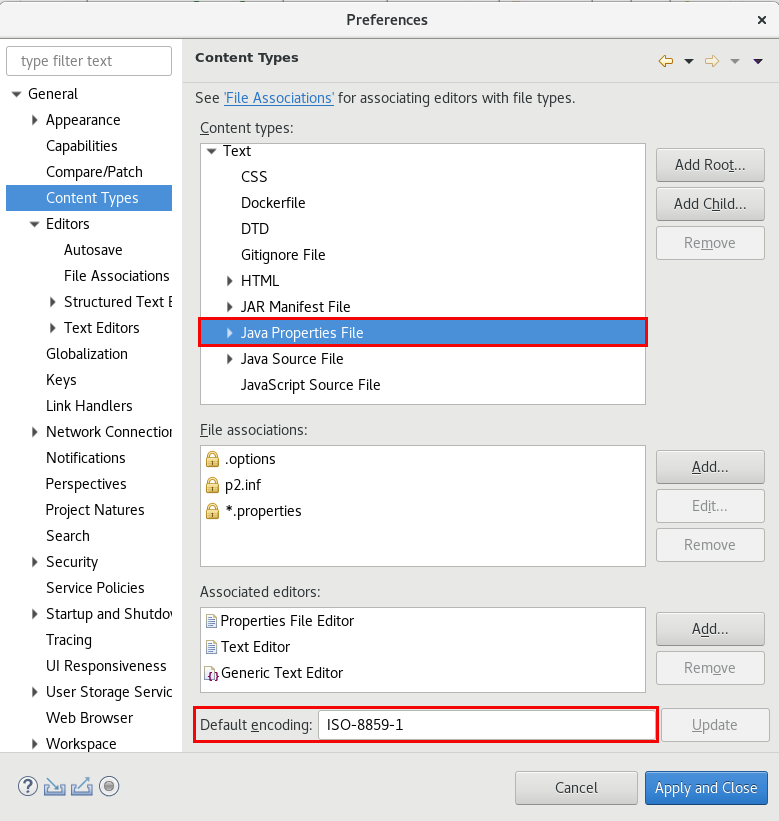
If you try to build a Maven project where UTF-8 is typically used the text in resource bundles will be broken. To change these settings you need to explicit set the default encoding to ‘UTF-8’ and press the ‘Update’ button right to this input field:
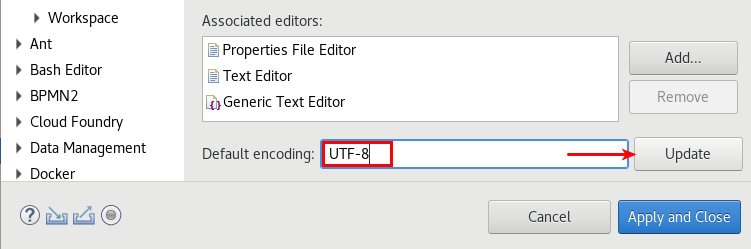
I recommend to set the default encoding for Java Properties Files UTF-8.
Wildfly – Broken pipe (Write failed)
We at Imixs are running JBoss/Wildfly as an application server for our Web or Rest Services. Today I stumbled into a problem when I tried to post large data via a Jax-rs POST request. The exception I saw on the server log was at first not very meaning full:
imixsarchiveservice_1 | 18:50:21,531 INFO [org.apache.http.impl.execchain.RetryExec] (EJB default - 2) I/O exception (java.net.SocketException) caught when processing request to {}->http://imixsofficeworkflow:8080: Broken pipe (Write failed)
imixsarchiveservice_1 | 18:50:21,531 INFO [org.apache.http.impl.execchain.RetryExec] (EJB default - 2) Retrying request to {}->http://imixsofficeworkflow:8080
imixsarchiveservice_1 | 18:50:21,537 INFO [org.apache.http.impl.execchain.RetryExec] (EJB default - 2) I/O exception (java.net.SocketException) caught when processing request to {}->http://imixsofficeworkflow:8080: Broken pipe (Write failed)
imixsarchiveservice_1 | 18:50:21,537 INFO [org.apache.http.impl.execchain.RetryExec] (EJB default - 2) Retrying request to {}->http://imixsofficeworkflow:8080
imixsarchiveservice_1 | 18:50:21,545 INFO [org.apache.http.impl.execchain.RetryExec] (EJB default - 2) I/O exception (java.net.SocketException) caught when processing request to {}->http://imixsofficeworkflow:8080: Broken pipe (Write failed)
imixsarchiveservice_1 | 18:50:21,545 INFO [org.apache.http.impl.execchain.RetryExec] (EJB default - 2) Retrying request to {}->http://imixsofficeworkflow:8080
My corresponding jax-rs implementation was quite simple:
@POST @Produces(MediaType.APPLICATION_XML) @Consumes({ MediaType.APPLICATION_XML, MediaType.TEXT_XML }) public Response postSnapshot(XMLDocument xmlworkitem) { try { ... } catch (Exception e) { e.printStackTrace(); return Response.status(Response.Status.NOT_ACCEPTABLE).build(); } }
The data object ‘xmlworkitem’ in my case is a XML Stream containing more than 24mb of data.
The Problem
After some research I found that the reason for my problem was the default http server configured in Wildfly standalone.xml. The default configuration looks like this:
<server name=”default-server”>
<http-listener name=”default” socket-binding=”http” redirect-socket=”https” enable-http2=”true”/>
…..
</server>
What you can’t see is that there is a a default max-post-size of 25485760 (25mb). But of course you can overwrite this setting by adding the attribute max-post-size with a maximum of e.g. 100mb:
<server name=”default-server”>
<http-listener name=”default” max-post-size=”104857600″ socket-binding=”http” redirect-socket=”https” enable-http2=”true”/>
…..
</server>
Now my Rest Interface works as expected and accepts also large amount of Data.
Note: I do not use the Wildfly CLI tool to configure the server because I run my server as a docker container with a pre configured standalone.xml file. But you can see the solution also in the Wilfly Forum.
Don’t Miss Eclipse Photon Update 2018-12 for Linux!
Eclipse Photon, which was released 2018, provides a much better support for GTK on the Linux platform. This was one of the big features for Linux users and there was a lot of news about this feature in the summer 2018. We from Imixs-Workflow use Eclipse on Linux from the first days. Our BPMN modeling tool Imixs-BPMN is based on Eclipse and the Graphiti library, which is a graphical modeling framework within Eclipse. Before Eclipse Photon was released it was nearly impossible to run Eclipse in GTK-2 mode. But with Eclipse Photon this mode becomes deprecated and modeling works now also nice in GTK-3 default mode.
But it still was a little bit stuttering if you tried to model large diagrams:
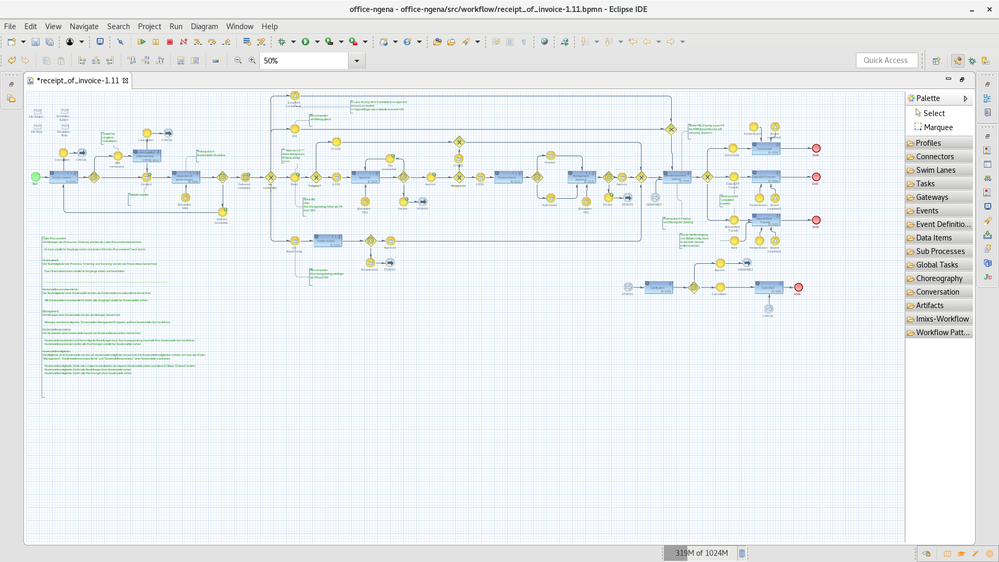
A little bit frustrated about that still bad behavior I installed today the Eclipse Photon Update 2018-12. (eclipse-jee-2018-12-R-linux-gtk-x86_64.tar.gz).
I didn’t expect any difference from my current Eclipse Photon installation which was already up-to-date. But the difference was so extremely surprising that I didn’t want to believe my eyes. The entire workspace is much faster and working with large models is now possible without delay and stuttering.
So do not miss the Eclipse Photon Update 2018-12R if you are working on Linux!
Monitoring Docker Swarm
In one of my last Blog Posts I explained how you can setup a Lightweight Docker Swarm Environment. The concept, which is already an open infrastructure project on Github enables you to run your business applications and microservices in a self-hosted platform.
Today I want to explain how you can monitor your Docker Swarm environment. Although Docker Swarm greatly simplifies the operation of business applications, monitoring is always a good idea. The following short tutorial shows how you can use Prometheus and Grafana to simplify monitoring.
Prometheus is a monitoring solution to collect metrics from several targets. Grafana is an open analytics and monitoring platform to visualize data collected by Prometheus.
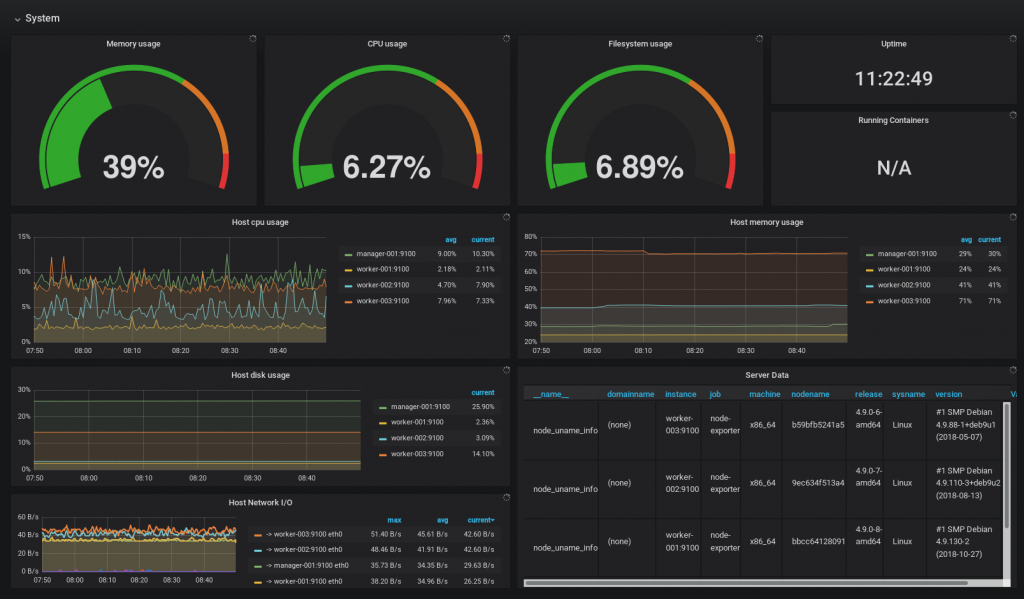
Both services can be easily integrated into Docker Swarm. There is no need to install extra software on your server nodes. You can simply start with a docker-compose.yml file to define your monitoring stack and a prometeus.yml file to define the scrape configuration. You can find the full concept explained here on Github in the Imixs-Cloud project.
Continue reading “Monitoring Docker Swarm”JSF Best Practice
I do developing with Java Server Pages (JSF) since the early beginning with version 1.0. Today , 17 years later, we have version 2.3 and thus a lot of improvements and new features. I’ve made many mistakes in using this standard web framework in my own applications. And therefore I would like to show some best practice rules in this Blog. And I hope this will simplify the work with JSF – for you and also for me – during refactoring my own applications. Most of which I write here comes from the great book ‘ The Definitive Guide to JSF in Java EE 8’ written by Bauke Scholtz (BalusC) and Arjan Tijms. I recommend you to buy this book if you have had already made experience with JSF. So let’s start…
Continue reading “JSF Best Practice”JSF, f:ajax and render…..
Designing JSF pages with the usage of the <f:ajax> tag can be a pretty frustrating affair. For example, if you’re trying to build a table with Ajax behavior, it often won’t work the way you expect it to. For example imaging a table with a delete-button which changes the content of your table:
<h:panelGroup layout="block" id="artikel_table_id" binding="#{artikelTableContainer}"> <table> <ui:repeat var="bookingItem" value="#{childItemController.childItems}"> <tr> <td>....</td> <td><h:commandLink value="delete" actionListener="#{childItemController.remove(bookingItem.item['numpos'])}"> <f:ajax render="artikel_table_id"/> </h:commandLink> </td> </tr> </ui:repeat> </table> ....
This example won’t work even if it looks logical. The problem here is the render attribute pointing to the id of an outer h:panelGroup. This UI component tree is created during the initial build of the view. If You’re manipulating the component references after building the view, the changes will not be reflected in the UI component tree during a ajax event life-cycle if you do not care to also execute the compete tree.
To solve this you can change the render attribute in the following way:
<f:ajax render="#{artikelTableContainer.clientId}" execute="#{artikelTableContainer.clientId}"/>
In this way now you have a binding to a component instance which forces jsf to execute and to rebuild the complete UI component tree. Note: the execution is necessary to update input fields placed within a table row.
ui:repeat versus c:forEach
At a first look it seems that between ui:repeat and c:forEach there is not big difference. This is true if only want to visualize a data set. But if you want to manipulate the entries too – e.g in a table with an embedded edit-mode than you can run in trouble if you are working with an ui:repeat. As explained in the example before, the elements inside a ui:repeat are not part of the component tree. This becomes an issue if your entries are editable. It will work on the first look if you simply submit the outer component. But in case of a JSF validation error, which is initiating the JSF-Life-Cycle the component tree will lost your entered values.
For that reason it is recommended to use a c:forEach tag instead of a ui:repeat if your data set is editable.
Enclosing Ajax Tags
If you use an enclosing ajax tag like in the following example, please note that you need to use a c:foreach instead of ui:repeat :
<h:panelGroup binding="#{artikelTableContainer}"> <f:ajax onevent="updateOrderItems"> <table> <c:forEach var="orderitem" items="#{childItemController.childItems}"> <tr> <td>....</td> <td><h:commandLink value="delete" actionListener="#{childItemController.remove(bookingItem.item['numpos'])}"> <f:ajax render="#{artikelTableContainer.clientId}" execute="#{artikelTableContainer.clientId}"/> </h:commandLink> </td> </tr> </c:forEach> </table> </f:ajax> </h:panelGroup>
In this case you should not call the ‘execute’ in the enclosing ajax!